Tutorial
|
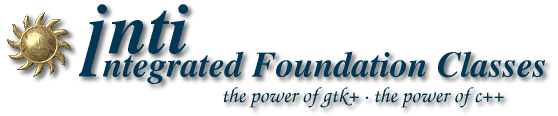
|
|
|
Dialogs
The Dialog widget is very simple, and is actually
just a window with a few things pre-packed into it for you. Simply, it
creates a window, and then packs a vertical box called the
"client_area"
into the top, which contains a separator and then a horizontal box
called the "action_area".
The Dialog widget can be used for pop-up messages to the user, and
other similar tasks. There are three constructors to create a new
Dialog.
Dialog();
Dialog(const String& title,
Gtk::Window
*parent = 0, Gtk::DialogFlagsField flags =
Gtk::DIALOG_DESTROY_WITH_PARENT);
Dialog(const String& title,
Gtk::Window
*parent, Gtk::DialogFlagsField flags, const char*first_button_text,
...);
|
The first constructor will create an empty dialog,
leaving it up to you to use it. You could pack a button in the
action_area by doing something like this:
Gtk::Button *button = new Gtk::Button("OK");
dialog->action_area()->pack_start(*button);
button->show();
|
And you could add to the client_area by packing, for instance, a label
in it, try something like this:
Gtk::Label *label = new Gtk::Dialog("Dialogs are groovy");
dialog->client_area()->pack_start (*label);
label->show();
|
As an example in using the dialog box, you could
put two buttons in the action_area, a Cancel button and an Ok button,
and a label in the client_area, asking the user a question or giving an
error etc. Then you could attach a different signal to each of the
buttons and perform the operation the user selects.
If the simple functionality provided by the default vertical and
horizontal boxes in the two areas doesn't give you enough control for
your application, then you can simply pack another layout widget into
the boxes provided. For example, you could pack a table into the
client_area.
The last two constructors are for convenience and let you set the title
for the dialog box, the parent if the dialog box is modal,
and
one or more dialog flags:
- DIALOG_MODAL - make the dialog modal
- DIALOG_DESTROY_WITH_PARENT - ensures that the dialog window is
destroyed together with the specified parent.
- DIALOG_NO_SEPARATOR - omits the separator between the client_area
and the action_area.
After the flags argument the last constructor
takes a variable argument list of button text/response ID pairs, with a
null pointer ending the list. Button text can be either a stock ID such
as GTK_STOCK_OK, or some arbitrary text but must be a C-style character
string, not a String object. A response ID can be any positive number,
or one of the values in the Gtk::ResponseType enumeration.
- RESPONSE_NONE
- RESPONSE_REJECT
- RESPONSE_ACCEPT
- RESPONSE_DELETE_EVENT
- RESPONSE_OK
- RESPONSE_CANCEL
- RESPONSE_CLOSE
- RESPONSE_YES
- RESPONSE_NO
- RESPONSE_APPLY
- RESPONSE_HELP
If the user clicks one of these dialog buttons,
Gtk::Dialog will emit the response signal with the
corresponding response ID. If a Gtk::Dialog receives the delete_event
signal, it will emit response with a response
ID
of Gtk::RESPONSE_DELETE_EVENT. However, destroying a dialog does not
emit the response signal; so be careful relying on response
when using the Gtk::DIALOG_DESTROY_WITH_PARENT flag. Buttons
are from left to right, so the first button in the list will be the
left
most button in the dialog.
You can use one the following methods to either add a single button or
several buttons at once using a variable argument list of button
text/response ID pairs, as in the last constructor.
Gtk::Button* add_button(const char *button_text, int
response_id);
void add_buttons(const
char *first_button_text, ...); |
The easiest way to display a modal dialog is to let Gtk::Dialog do it
for you by calling its run() method:
This blocks in a recursive main loop until the
dialog either emits the response signal, or is destroyed. If
the dialog is destroyed, Gtk::Dialog::run() returns Gtk::RESPONSE_NONE.
Otherwise, it returns the response ID from the response
signal
emission. Before entering the recursive main
loop, Gtk::Dialog::run() calls Gtk::Widget::show() on the dialog
for you. Note that you still need to show any children of the dialog
yourself.
During Gtk::Dialog::run(), the default behavior of delete_event is
disabled; if the dialog receives delete_event, it will not be
destroyed as windows usually are, and Gtk::Dialog::run() will return
Gtk::RESPONSE_DELETE_EVENT. Also, during Gtk::Dialog::run() the dialog
will be modal. You can force Gtk::Dialog::run() to return at any time
by
calling Gtk::Dialog::response() to emit the response signal.
Destroying the dialog during Gtk::Dialog::run() is a very bad idea,
because your post-run code won't know whether the dialog was destroyed
or not.
After Gtk::Dialog::run() returns, you are responsible
for hiding or destroying the dialog if you wish to do so.
Typical usage of this method might be:
int
result = dialog->run();
switch (result)
{
case RESPONSE_ACCEPT:
do_application_specific_something();
break;
default:
do_nothing_since_dialog_was_canceled();
break;
}
dialog->dispose(); |
You can emit the response signal with a specified response ID by
calling:
void
response(int response_id);
|
This method is used to indicate that the user has
responded to the dialog in some way; typically either you or
Gtk::Dialog::run() will be monitoring the response signal and
take appropriate action.
void
set_default_response(int response_id);
|
Sets the last widget in the dialog's action area
with the given response_id as the default widget for the dialog.
Pressing "Enter" normally activates the default widget.
void
set_response_sensitive(int response_id, bool setting);
|
Calls Gtk::Widget::set_sensitive(setting) for each
widget in the dialog's action area with the given response_id. A
convenient way to sensitize/desensitize dialog buttons.