Tutorial
|
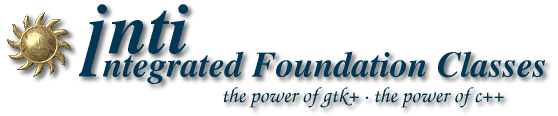
|
|
|
Frames
Frames can be used to enclose one or a group of
widgets with a box which can optionally be labeled. The position of the
label and the style of the box can be altered to suit.
A Frame can be created with one of the following constructors:
explicit
Frame(Gtk::ShadowType type = Gtk::SHADOW_ETCHED_IN);
explicit Frame(const
String& label, Gtk::ShadowType type = Gtk::SHADOW_ETCHED_IN); |
The first constructor creates a Frame with the
specified ShadowType, which by default is SHADOW_ETCHED_IN. The second
constructor additionally lets you specify a label for the frame. The
label is by default placed in the upper left hand corner of the frame.
The text of the label can be changed using the next method:
void
set_label(const String& label);
|
A label widget can be added later by either
calling the above method, in which case a label widget is created for
you, or by specifically calling the next method followed by a call to
Gtk::Frame::set_label() to set the label text.
void
set_label_widget(Widget& label_widget);
|
The position of the label can be changed using this method:
void
set_label_align(float xalign, float yalign);
|
The xalign and yalign
arguments
take values between 0.0 and 1.0. xalign indicates the
position
of the label along the top horizontal of the frame. yalign
indicates the vertical position with respect to the top horizontal of
the frame - either above (0.0), below (1.0) or somewhere over it. The
default value of xalign is 0.0 which places the label at the left hand
end of the frame. The default value of yalign is 0.5 which places the
middle of the label over the top horizontal of the frame.
The next function alters the style of the box that is used to outline
the frame.
void
set_shadow_type(Gtk::ShadowType type);
|
The type argument can take one of the following values from
the Gtk::ShadowType enumeration:
- SHADOW_NONE
- SHADOW_IN
- SHADOW_OUT
- SHADOW_ETCHED_IN - the default
- SHADOW_ETCHED_OUT
The following code example illustrates the use of the Frame widget.
The header file for Frame Example is
frame.h:
#include<inti/main.h>
#include <inti/core.h>
using namespace Inti;
class FrameWindow : public Gtk::Window
{
public:
FrameWindow();
virtual ~FrameWindow();
};
|
and the source file is frame.cc:
#include"frame.h"
#include <inti/gtk/frame.h>
FrameWindow::FrameWindow()
{
set_title("Frame Example");
set_size_request(300, 300);
// Sets the border width
of the window.
set_border_width(10);
// Create a Frame
Gtk::Frame *frame = new Gtk::Frame;
add(*frame);
// Set the frame's label
frame->set_label("Inti Frame
Widget");
// Align the label at
the right of the frame
frame->set_label_align(1.0, 0.0);
// Set the style of the
frame
frame->set_shadow_type(Gtk::SHADOW_ETCHED_OUT);
frame->show();
}
FrameWindow::~FrameWindow()
{
}
INTI_MAIN(FrameWindow)
|
The INTI_MAIN macro is a convenience macro that
writes a simple main function, its only argument is the name of the
main
window class. The macro is defined in <inti/main.h>
as:
#define
INTI_MAIN(MainWidget)\
int main (int argc, char *argv[])\
{\
Inti::Main::init(&argc,
&argv);\
MainWidget main_widget;\
main_widget.sig_destroy().connect(slot(&Inti::Main::quit));\
main_widget.show();\
Inti::Main::run();\
return 0;\
} |
Most main functions in C++ are as simple as this
because all the creation work for the main window is done inside its
constructor, not the main function.