Tutorial
|
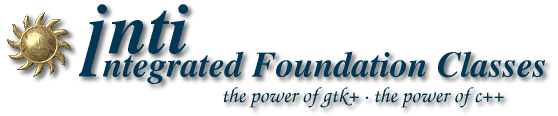
|
|
|
Creating the View Component
While there are several different models to choose
from, there is only one view widget to deal with. It works with either
the list or the tree store. Setting up a TreeView is not a difficult
matter. It needs a TreeModel to know where to retrieve its data from.
You can create a TreeView with one of the following constructors:
TreeView();
explicit
TreeView(Gtk::TreeModel&
model); |
You can set and get the model for the TreeView by calling:
void
set_model(Gtk::TreeModel *model);
Gtk::TreeModel* get_model() const;
|
Once the TreeView widget has a model, it will need
to know how to display the model. It does this with columns and cell
renderers.
Cell renderers are used to draw the data in the tree
model in a way. There are three cell renderers that come with Inti.
They
are the CellRendererText, CellRendererPixbuf and CellRendererToggle. It
is relatively easy to write a custom renderer.
The constructors for the three cell renderers are simple:
CellRendererPixbuf();
CellRendererText();
CellRendererToggle();
|
A TreeViewColumn is the object
that TreeView uses to organize the vertical columns in the tree view.
It
needs to know the name of the column to label for the user, what type
of
cell renderer to use, and which piece of data to retrieve from the
model
for a given row.
You can create a TreeViewColumn by calling one of the following
constructors:
TreeViewColumn();
TreeViewColumn(const
String& title, Gtk::CellRenderer *cell, ...);
TreeViewColumn(const String& title,
Gtk::CellRenderer& cell, const
std::map<int, String>&
attributes); |
The title is the title of the tree
column
and the cell is the cell to pack into the beginning of the
tree column. The variable argument list is a null terminated list of
tree column attributes to set. These should be in
attribute(char*)/column(number)pairs. The last constructor takes a
std::map of column(number)/attribute(String) pairs, the order being
reversed because the column number is the key and the key must come
first.
For example, to create a column using a list char*/column pairs you
would do this:
Gtk::CellRenderer
*renderer = new Gtk::CellRendererText;
Gtk::TreeViewColumn *column = new
Gtk::TreeViewColumn ("Author", renderer, "text",
AUTHOR_COLUMN, 0);
tree_view->append_column(*column);
|
and creating a column using a std::map<
int, String> you would do
this:
#include <map>
Gtk::CellRenderer *renderer = new
Gtk::CellRendererText;
std::map<int, String>
attributes;
attributes.insert(std::pair<int,
String>(AUTHOR_COLUMN, "text"));
Gtk::TreeViewColumn *column = new
Gtk::TreeViewColumn("Author", *renderer, attributes);
|
At this point, all the steps in creating a displayable tree have been
covered. The model is created, data is stored in it, a tree view is
created and columns are added to it.
Most applications will need to not only deal with displaying data, but
also receiving input events from users. To do this, simply get a
reference to a selection object and connect to the "changed" signal.
//
Setup the selection handler
Gtk::TreeSelection *selection =
tree_view->get_selection();
selection->set_mode (Gtk::SELECTION_SINGLE);
selection->sig_changed().connect(slot(this,
&MyClass::changed_handler));
|
where the changed_handler has the prototype:
void
MyClass::changed_handler();
|
Then to retrieve data for the row selected:
void
MyClass::changed_handler()
{
Gtk::TreeModel *model = 0;
Gtk::TreeIter iter;
if
(selection->get_selected(&model, &iter))
{
String author;
model->get_value(iter,
AUTHOR_COLUMN, author);
g_print ("You selected a book by
%s\n", author);
}
}
|