Tutorial
|
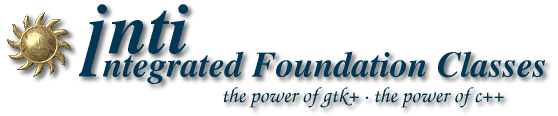
|
|
|
Images
The Image widget displays an image. Various kinds
of objects can be displayed as an image; most typically, you would load
a Gdk::Pixbuf ("pixel buffer") from a file, and then display that.
There's a convenience constructor to do this:
explicit
Image(const String& filename); |
This creates a new Image displaying the file filename.
If the file isn't found or can't be loaded, the resulting Image will
display a "broken image" icon. This constructor always creates a valid
Image widget.
If the file contains an animation, the image will contain an animation.
If you need to detect failures to load the file, use Gdk::PixbufLoader
to load the file yourself, then create the Image from the pixbuf.
Alternatively, you can construct an empty image:
and then call the following set method:
void
set(const String& filename); |
Image is derived from Gtk::Misc, which implies
that you can align it (center, left, right) and add padding to it,
using
Gtk::Misc methods.
Image is a no window widget (has no Gdk::Window of its own),
so by default does not receive events. If you want to receive events on
the image, such as button clicks, place the image inside an EventBox, then connect to the event signals
on the event box.
There are 7 other Image constructors, each with a corresponding set
method so we'll look at them together.
explicit
Image(Gdk::Pixbuf *pixbuf);
void set(Gdk::Pixbuf *pixbuf);
|
The above constructor and set() method creates a
new Image displaying pixbuf. The Image does not assume a reference to
the pixbuf; you still need to unref it if you own references. Image
will
add its own reference rather than adopting yours. Note that the Image
created will not react to state changes. Should you want that, you
should use a Gtk::IconSet.
Image(Gtk::IconSet
*icon_set, Gtk::IconSize size) ;
void set(Gtk::IconSet *icon_set,
Gtk::IconSize size);
|
The above constructor and set() method creates an
Image displaying an icon set. The image
size can be one of
the
following values in the Gtk::IconSize enumeration:
- ICON_SIZE_MENU
- ICON_SIZE_SMALL_TOOLBAR
- ICON_SIZE_LARGE_TOOLBAR
- ICON_SIZE_BUTTON
- ICON_SIZE_DND
- ICON_SIZE_DIALOG
Usually it's better to create a Gtk::IconFactory, put your icon sets in
the icon factory, add the icon factory to the list of default factories
with Gtk::IconFactory::add_default(), and then use stock image
constructor or stock set() method. This will allow themes to override
the icon you ship with your application.
The Image does not assume a reference to the icon set; you still need
to unref it if you own references. Image will add its own reference
rather than adopting yours.
Image(const char *stock_id, Gtk::IconSize size);
void set(const
char *stock_id, Gtk::IconSize size);
|
The above constructor and set() method creates an
Image displaying a stock icon. Sample stock icon names are
GTK_STOCK_OPEN, GTK_STOCK_EXIT etc, and are defined in
<gtk/gtkstock.h>. If the stock icon name isn't known, a "broken
image" icon will be displayed instead. You can register your own stock
icon names, see the Gtk::IconFactory methods add_default() and add().
Image(Gdk::Pixmap
*pixmap, Gdk::Bitmap *mask);
void set(Gdk::Pixmap *pixmap,
Gdk::Bitmap *mask);
|
The above constructor and set() method creates an
Image widget displaying a pixmap with a mask. The Image does not assume
a reference to the pixmap or mask; you still need to unref them if you
own references. Image will add its own reference rather than adopting
yours.
Image(Gdk::Image *image,
Gdk::Bitmap *mask);
void set(Gdk::Image *image,
Gdk::Bitmap *mask);
|
The above constructor and set() method creates an
Image widget displaying a image with a mask. A Gdk::Image is a
client-side image buffer in the pixel format of the current display.
The
Image does not assume a reference to the image or mask; you still need
to unref them if you own references. Image will add its own reference
rather than adopting yours.
explicit
Image(Gdk::PixbufAnimation& animation);
void set(Gdk::PixbufAnimation&
animation);
|
The above constructor and set() method creates an
Image displaying the given animation. The Image does not assume a
reference to the animation; you still need to unref it if you own
references. Image will add its own reference rather than adopting yours.
explicit
Image(const char **xpm_data); |
The above convenience constructor creates a new
pixbuf by parsing XPM data in memory. This data is commonly the result
of including an XPM file into a program's source. It then calls the
pixbuf set() method above to create the image.
Gtk::ImageType
get_storage_type() const; |
The get_storage_type() method returns the image
storage type and can be one of the following values in the
Gtk::ImageType enumeration:
- IMAGE_EMPTY
- IMAGE_PIXMAP
- IMAGE_IMAGE
- IMAGE_PIXBUF
- IMAGE_STOCK
- IMAGE_ICON_SET
- IMAGE_ANIMATION
For a good example on how to load and display images see the image demo
file "image.cc" in the "demo/inti-demo" source directory.