Tutorial
|
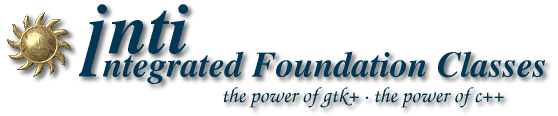
|
|
|
AccelLabel
The AccelLabel widget is derived from Label and
displays an accelerator key on the right of the label text, e.g.
'Ctl+S'. It is commonly used in menus to show the keyboard short-cuts
for commands. You most probably wont have much use for an AccelLabel in
your day to day programming but if you ever need a custom menu item you
will.
You can create an AccelLabel by calling one of the following
constructors:
AccelLabel();
explicit AccelLabel(const String& str, bool
use_underline = false); |
The first constructor creates an empty AccelLabel.
The second constructor creates an AccelLabel that displays the
specified
character string. If use_underline is true the
string
is parsed for an underscore preceding the mnemonic character.
After construction you can change the label's text by calling one of
the following Gtk::Label methods:
void
set_text(const String& str);
void set_text_with_mnemonic(const String& str); |
The sole argument is the new string to display.
The second method, set_text_with_mnemonic() parses the string for the
mnemonic character (i.e. the character preceded by an underscore). The
space needed for the new string will be automatically adjusted if
needed.
The accelerator key to display is not set
explicitly. Instead, the AccelLabel displays the accelerators which
have
been added to a particular widget. This widget is set by calling the
following method:
void
set_accel_widget(Gtk::Widget& accel_widget);
|
For example, a MenuItem widget may have an
accelerator added to emit the "activate" signal when the 'Ctl+S' key
combination is pressed. An AccelLabel is created and added to the
MenuItem, and Gtk::AccelLabel::set_accel_widget() is called with the
MenuItem as its argument. The AccelLabel will now display 'Ctl+S' after
its label. Note that creating a MenuItem with a label (or one of the
similar functions for CheckMenuItem and RadioMenuItem) automatically
adds an AccelLabel to the MenuItem and calls
Gtk::AccelLabel::set_accel_widget() to set it up for you. So as I
said, you probably wont have much use for an AccelLabel unless
your are creating the menu item yourself.
An AccelLabel will only display accelerators which have
Gtk::ACCEL_VISIBLE set (see Gtk::AccelFlags). An AccelLabel can display
multiple accelerators and even signal names, though it is almost always
used to display just one accelerator key.
If you have a window with a menu, to create a
simple
menu item with an accelerator key you would do something like this:
//
Create a Gtk::AccelGroup and add it to the window.
Gtk::AccelGroup *accel_group = new
Gtk::AccelGroup;
window->add_accel_group(accel_group);
// Create the menu item using the
convenience function.
Gtk::MenuItem *save_item = new Gtk::MenuItem
("Save");
save_item->show();
menu->add (*save_item);
// Now add the accelerator to the
Gtk::MenuItem.
// Note Gtk::ACCEL_VISIBLE is the default and doesn't need to be
specified.
save_item->add_accelerator ("activate", *accel_group,
Gtk::AccelKey(GDK_s, Gdk::CONTROL_MASK));
|